I'm using ReactiveSearch (great Search UI library!) and am trying to figure out how I can just show a ample of a text article in the ReactiveList search results. Currently it shows the whole article but I just want the first 250 words or so to be displayed.
I just want say the first 250 words of that. Is that possible with ReactiveList? If so, how can I go about doing that or is there a feature o setting that I'm missing with ReactiveSearch?
This is the simple line that displays it currently:
<p className="card-text">{data.Text}</p>
It is possible to truncate the text after a few lines. But ReactiveSearch doesn't support this feature on the library level.
You can achieve this by using the package called react-truncate. You can read the documentation here.
Hope this helps!
Related
I've looked through the mermaid sequence diagram docs and found how to add links to actors in a sequence diagram, but I'm interested in adding links to a note.
I found that <br> is supported in notes but other tags are not. Is this possible?
sequenceDiagram
participant Alice
participant John
links Alice: {"Dashboard": "https://dashboard.contoso.com/alice", "Wiki": "https://wiki.contoso.com/alice"}
links John: {"Dashboard": "https://dashboard.contoso.com/john", "Wiki": "https://wiki.contoso.com/john"}
Alice->>John: Hello John, how are you?
John-->>Alice: Great!
Alice-)John: See you later!
Note over Alice, John: I want to<br>be a link
This may relate to your question: Try this chart below - if you click it, you will be redirected to Google.
The trick here is to combine the image and link functions in markdown.
[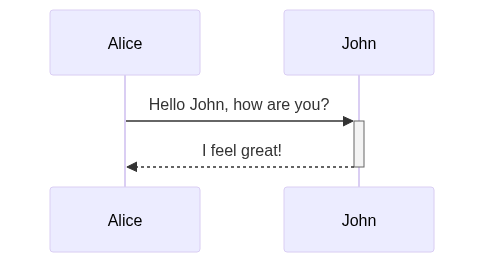](https://mermaid.live/edit#pako:eNo1j8EKwjAQRH9l3avtD-RQETyo4MlrLksytYU00TRBSum_m7a4p2F4w87MbIIFKx7xyfAGl15eUQbtqdzZ9QZ10xzvofOKrnAu0Kor6sKXJIKmkE87vPp1gestpehGLeDoFSHpwBUPiIP0tvya14Dm1GGAZlWkRSvZJc3aLwWVnMJz8oZVihkV57eV9K_GqhU3Fhe2TyE-9v7bjOUHTYdELA)
While it is possible to add link (I have read about it in the mermaid.js book and will get back to you in the near future), but depends on the format of the output (.png, etc) you may lost this function after rendering. The only format that makes it possible is html. As:
Possible Values You Can Set securityLevel to: … loose: Mermaid will
allow HTML tags in text. The interactivity in the diagrams provides
support for click events.
Page 83, The Official Guide to Mermaid.js by Kunt Sveidqvist & Ashish Jain
I wanted to cluster sentences based on their context and extract common keywords from similar context sentences.
For example
1. I need to go to home
2. I am eating
3. He will be going home tomorrow
4. He is at restaurant
Sentences 1 and 3 will be similar with keyword like go and home and maybe it's synonyms like travel and house .
Pre existing API will be helpful like using IBM Watson somehow
This API actually is doing what you are exactly asking for (Clustering sentences + giving key-words):
http://www.rxnlp.com/api-reference/cluster-sentences-api-reference/
Unfortunately the algorithm used for clustering and the for generating the key-words is not available.
Hope this helps.
You can use RapidMiner with Text Processing Extension.
Insert each sentence in a seperate file and put them all in a folder.
Put the operators and make a design like below.
Click on the Process Documents from files operator and in the right bar side choose "Edit list" on "Text directories" field. Then choose the folder that contains your files.
Double click on Process Documents from files operator and in the new window add the operators like below design(just the ones you need).
Then run your process.
EZ stuff but after an hour.. =filter(May15!A:S , May15!E:E="Authorization") is yielding a rich populated sheet. However I can't get OR working! Despite it working elsewhere in the sheet. I'd like other possibilities via the same filter. I tried several including the OR this way
=filter(May15!A:S , OR(May15!E:E="Authorization" , May15!E:E="bigwhale", May15!E:E="hi"))
.. to no avail. Any help appreciated. Also, I read somewhere the OR could be accessed using a "+" and that sounded like a neat method.. Thanks!
One possible way is to use RegEx:
=filter(H:H , REGEXMATCH(E:E,JOIN("|",A1:A3)))
put in A1:A3:
Authorization
bigwhale
hi
This trick is useful when you need to add conditions, just paste one more value in cell A4 and use range A1:A4
Another way is to use plus sign:
=FILTER(H:H,(E:E="Authorization")+(E:E="bigwhale")+(E:E="hi"))
I am creating an application where I need to implement autocompletion when a user is typing into an text input, with the 10 nearest/highest ranking words appearing below the text field.
I've been given a fairly big list of around 80,000 words and their respective 'priority' - a number which determines how high up they appear in the autocomplete depending on the size of the number, like this:
"transport international";19205
"taxi";18462
"location de voitures";18160
"police";18126
"formation";17858
I am kinda new to iOS development and was wondering what is the best way to do this - should I split the 80,000 phrases into smaller files, or just keep it in one? What would be faster?
I have seen autocompletion used in an example for iOS but it was for a very small amount of suggestions - I haven't seen it done using a file this large before, and obviously I would like to make it as fast as possible for added user experience.
Any suggestions as to examples, tutorials or code suggestions would be greatly appreciated, thanks.
If you prefer something that does autocomplete but is a direct subclass of UITextField, then MLPAutoCompleteTextField may be of interest to you.
MLPAutoCompleteTextField works by simply asking its autocomplete datasource for an array of autocomplete suggestions each time the text in the textfield changes. It can even automatically sort words so that the ones closest to what the user is typing will appear at the top of the autocomplete list (using a Levenshtein Distance algorithm). Autocomplete suggestions can be simple strings, or objects that implement MLPAutoCompletionObject protocol.
Tip: For a large dataset of autocomplete terms, you'll probably want to break up your list based on starting letters. (Example: When the user enters the letter F, you give the autocomplete textfield only a list of words that start with F.)
MLPAutoCompleteTextField can efficiently sort several thousand suggestions in a reasonable amount of time, and will never block the UI while it sorts.
At the moment, weighted suggestions (that override the default sorting) aren't possible but it's a planned feature.
You may want to use this repo HTAutocompleteTextField, perfect solution.
https://github.com/TarasRoshko/TRAutocompleteView
Just conform TRAutocompleteItemsSource protocol and that's it. Protocol is designed with async support in mind. Demo app and sample TRGoogleMapsAutocompleteItemsSource should greatly help you with it.
This link worked well for me. Depending on your code, just don't miss the difference between UITextField and UITextView.
No extra libraries, just an easy custom UITableView and search function.
While we ask a question, SO shows related questions. If we hover on the questions the content is displayed as a tooltip which contains linebreaks, indentations etc. What technique SO uses for it? How to display tooltips wit the formatting?
Other than, jquery/javascript is there any simple way to achieve it?
Was just wondering, should i ask this on Meta?
It is just a title attribute on the hyperlink, plain and simple.
<a href="..." title="The value is approximately
3.14159265
but for simplicity you can always round it
to exactly 3.0">What is the value of Pi?</a>
In IE, Chrome & Safari, it honors the line breaks a bit more strictly than say Firefox or Opera.
I took this html actually from stackoverflow. Here is no magic here:
Here's a dumb question which I can't find an answer to:
I have a table which contains 20 fields, a few of which are date/time. I am interested in pulling all the fields. I would like to … ">Selecting fields in SQL Select statements (Dumbest SQL Question)</a>
As you see, title can have line breaks!